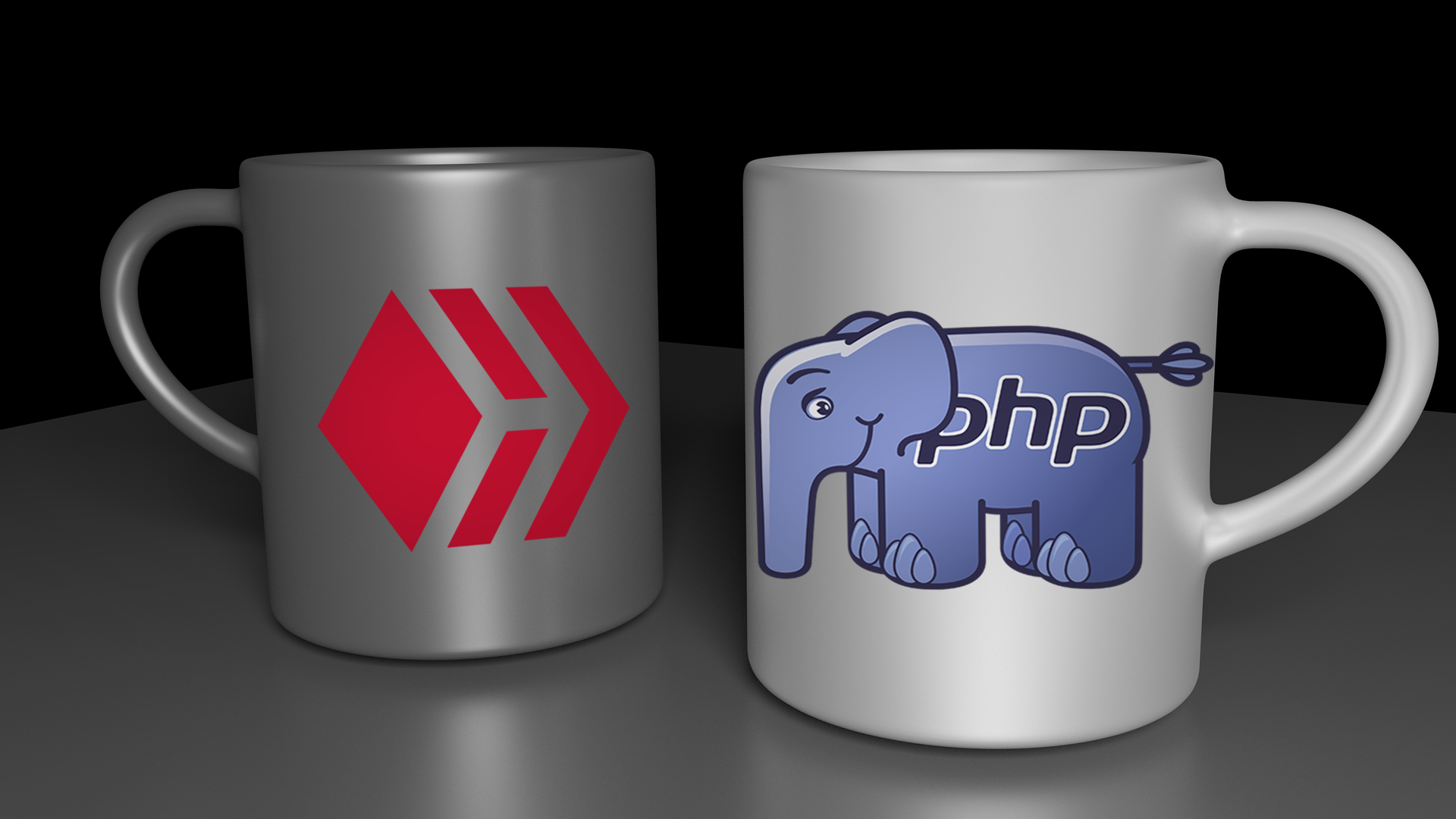
# Hive-PHP
This one took a while despite having some demand from PHP developers. But it is finally here.
The serialization and signing are done natively on PHP. Every line of code has been written by me.
I did not test all the operations but all the operations should work. Please do test if you can and let me know if there are any problems.
The documentation is available on both gitlab and packagist. But for the sake of keeping them on blockchain will put them here too.
***
### Installation
```
composer require mahdiyari/hive-php
```
### Initialization
```
$hive = new Hive($options?);
```
Example:
```
include 'vendor/autoload.php';
use Hive\Hive;
$hive = new Hive();
// or
// default options - these are already configured
$options = array(
'rpcNodes'=> [
'https://api.hive.blog',
'https://rpc.ausbit.dev',
'https://rpc.ecency.com',
'https://api.pharesim.me',
'https://api.deathwing.me'
],
'chainId'=> 'beeab0de00000000000000000000000000000000000000000000000000000000',
'timeout'=> 7
);
// Will try the next node after 7 seconds of waiting for response
// Or on a network failure
$hive = new Hive($options);
```
### Usage
### API calls:
```
$hive->call($method, $params);
```
Example:
```
$result = $hive->call('condenser_api.get_accounts', '[["mahdiyari"]]');
// returns the result as an array
echo $result[0]['name']; // "mahdiyari"
echo $result[0]['hbd_balance']; // "123456.000 HBD"
```
### Private Key:
```
$hive->privateKeyFrom($string);
$hive->privateKeyFromLogin($username, $password, $role);
```
Example:
```
$privateKey = $hive->privateKeyFrom('5JRaypasxMx1L97ZUX7YuC5Psb5EAbF821kkAGtBj7xCJFQcbLg');
// or
$privateKey = $hive->privateKeyFromLogin('username', 'hive password', 'role');
// role: "posting" or "active" or etc
echo $privateKey->stringKey; // 5JRaypasxMx1L97ZUX7YuC5Psb5EAbF821kkAGtBj7xCJFQcbLg
```
### Public Key:
```
$hive->publicKeyFrom($string);
$privateKey->createPublic()
```
Example:
```
$publicKey = $hive->publicKeyFrom('STM6aGPtxMUGnTPfKLSxdwCHbximSJxzrRjeQmwRW9BRCdrFotKLs');
// or
$publicKey = $privateKey->createPublic();
echo $publicKey->toString(); // STM6aGPtxMUGnTPfKLSxdwCHbximSJxzrRjeQmwRW9BRCdrFotKLs
```
### Signing
```
$privateKey->sign($hash256);
```
Example:
```
$message = hash('sha256', 'My super cool message to be signed');
$signature = $privateKey->sign($message);
echo $signature;
// 1f8e46aa5cbc215f82119e172e3dd73396ad0d2231619d3d71688eff73f2b83474084eb970955d1f1f9c2a7281681d138ca49fe90ac58bf069549afe961685d932
```
### Verifying
```
$publicKey->verify($hash256, $signature);
```
Example:
```
$verified = $publicKey->verify($message, $signature);
var_dump($verified); // bool(true)
```
### Transactions
There are two ways to broadcast a transaction.
#### Manual broadcast
Example:
```
$vote = new stdClass;
$vote->voter = 'guest123';
$vote->author = 'blocktrades';
$vote->permlink = '11th-update-of-2022-on-blocktrades-work-on-hive-software';
$vote->weight = 5000;
$op = array("vote", $vote);
// transaction built
$trx = $hive->createTransaction([$op]);
// transaction signed
$hive->signTransaction($trx, $privateKey);
// will return trx_id on success
$result = $hive->broadcastTransaction($trx);
var_dump($result);
// array(1) {
// 'trx_id' =>
// string(40) "2062bb47ed0c4c001843058129470fe5a8211735"
// }
```
#### Inline broadcast
Example:
```
$result = $hive->broadcast($privateKey, 'vote', ['guest123', 'blocktrades', '11th-update-of-2022-on-blocktrades-work-on-hive-software', 5000]);
var_dump($result);
// array(1) {
// 'trx_id' =>
// string(40) "2062bb47ed0c4c001843058129470fe5a8211735"
// }
```
### Notes for Transactions
Operations are in the following format when broadcasting manually:
```
array('operation_name', object(operation_params))
```
example:
```
$vote = new stdClass;
$vote->voter = 'guest123';
$vote->author = 'blocktrades';
$vote->permlink = '11th-update-of-2022-on-blocktrades-work-on-hive-software';
$vote->weight = 5000;
$op = array("vote", $vote);
```
Any parameter in opreation that is JSON (not to be confused with json strings), should be passed as an object.
Example:
The `beneficiaries` field in `comment_options` is in JSON format like this:
```
$beneficiaries = '[0, {"beneficiaries": [{"account": "mahdiyari","weight": 10000}]}]';
```
We should convert that into an object.
```
$beneficiaries = json_decode($beneficiaries);
```
(beneficiaries go inside the extensions)
```
$result = $hive->broadcast($privateKey, 'comment_options', ['author', 'permlink', '1000000.000 HBD', 10000, true, true, [$beneficiaries]]);
```
***
### How to know the operation parameters
Easiest way is to find the operation on a block explorer.
e.g. https://hiveblocks.com/tx/2062bb47ed0c4c001843058129470fe5a8211735
You can also search the operation in `/lib/Helpers/Serializer.php` to get an idea of what parameters it requires.
***
### Any missing features?
Create an issue or reach out to me.
***
### License
MIT
***
I hope PHP developers enjoy this and get away from the "hacks" they used so far to interact with Hive blockchain.
Composer package: https://packagist.org/packages/mahdiyari/hive-php
Gitlab repository: https://gitlab.com/mahdiyari/hive-php
***
Almost forgot the bonus content!
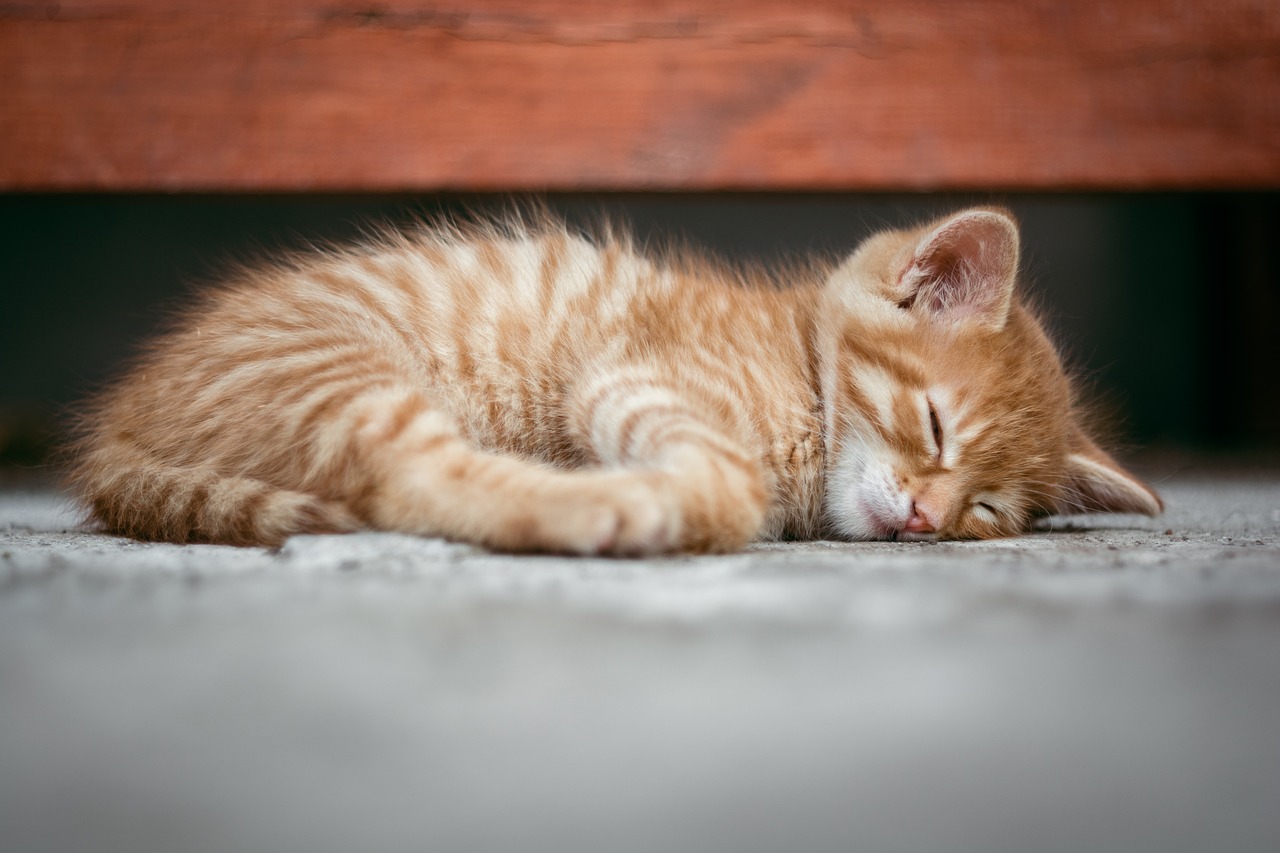
Made with ❤️ by @mahdiyari
Image source: pixabay.com
See: Hive-PHP - A real PHP library for Hive
by
@mahdiyari