JavaScript library for Ledger Nano S HIVE application
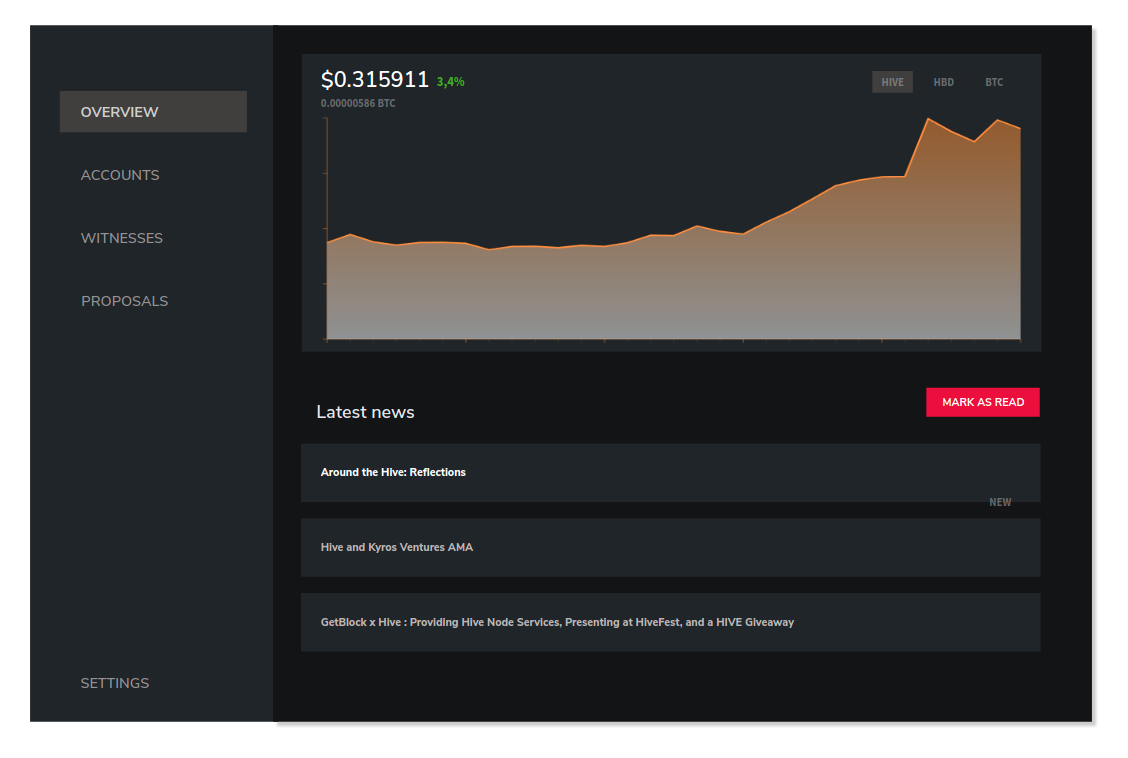
It's been a couple of months since @netuoso [published the initial version of the Hive Ledger App](https://hive.blog/hive-139531/@netuoso/hive-application-for-the-ledger-nano-s-x-hardware-wallet). In order to get official support from Ledger (i.e. the possibility to install it from Ledger Live), we need to meet several requirements. And I'm trying to fulfill them all so we could benefit from the hardware wallet support.
# Code review
First, we need to make sure that the application is stable and secure. I already made a preliminary review of the app and created a Pull Request with some fixes. The next step will be to review the code from a security perspective, following the guidelines provided by Ledger. This requires a bit more work and knowledge than validating transactions serialization etc, but we will get there.
* https://github.com/netuoso/ledger-app-hive/pull/5/
# Javascript library
To simplify communication with the ledger app, I created a JavaScript/Typescript library. It's really easy to use it with NodeJS. The library covers every functionality supported by the app.
Example usage:
```typescript
import Hive from '@engrave/ledger-app-hive';
import TransportNodeHid from '@ledgerhq/hw-transport-node-hid';
import Transport from '@ledgerhq/hw-transport';
( async () => {
console.log('Unlock your ledger....');
const transport = await TransportNodeHid.create();
console.log(`Established connection with Ledger Nano S`);
try {
const hive = new Hive(transport);
const {version, arbitraryData} = await hive.getAppConfiguration();
console.log("Current version:", version);
console.log("Arbitary data enabled:", arbitraryData);
const publicKey = await hive.getPublicKey(`48'/13'/0'/0'/0'`);
console.log("Public key:", publicKey);
} catch (e) {
console.error(e);
}
finally {
transport.close();
}
})();
```
## API
* [getAppConfiguration](#getappconfiguration)
* [getPublicKey](#getpublickey)
* [signTransaction](#signtransaction)
### getAppConfiguration
Get current configuration for HIVE App installed on a Ledger.
```typescript
async getAppConfiguration(): Promise<{version: string, arbitraryData: boolean}>
```
### getPublicKey
Retrieve public key from specified BIP32 path. You can set an additional parameter `confirm: boolean` which will ask the user to confirm the Public Key on his Ledger before returning it.
```typescript
async getPublicKey(path: string, confirm?: boolean): Promise<{publicKey: string}> {
```
### signTransaction
Sign basic transactions with the specified key path. This method will return signed transactions (a transaction with additional `signatures` property). Please note that extensions are not yet supported for most transactions and operations (except `beneficiaries` for `comment_options`).
```typescript
async signTransaction(tx: Transaction, path: string): Promise
```
Please be aware that the current implementation of the HIVE Ledger App does not support multisig. This could be also a cool feature and we might add it someday. For now, let's focus on publishing it.
The library is open-source and available on Gitlab and NPM:
* https://gitlab.com/engrave/ledger/ledger-app-hive
# Ledger Companion App
To prove that the application is working correctly, Ledger requires a sample Companion App.
I prepared an initial design for a new wallet app and will start developing it soon. The plan is to make it as a wallet targeted at large stakeholders willing to invest in HIVE. It will be focused on governance (voting for witnesses and proposals) and the most significant chain news.
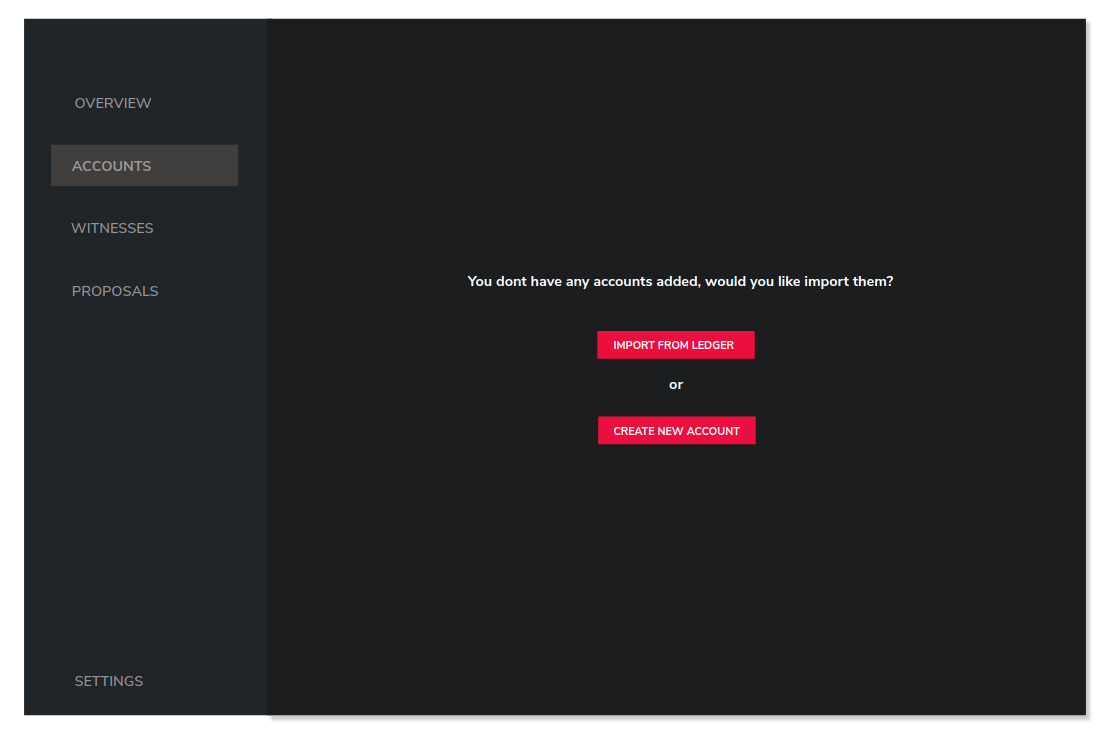
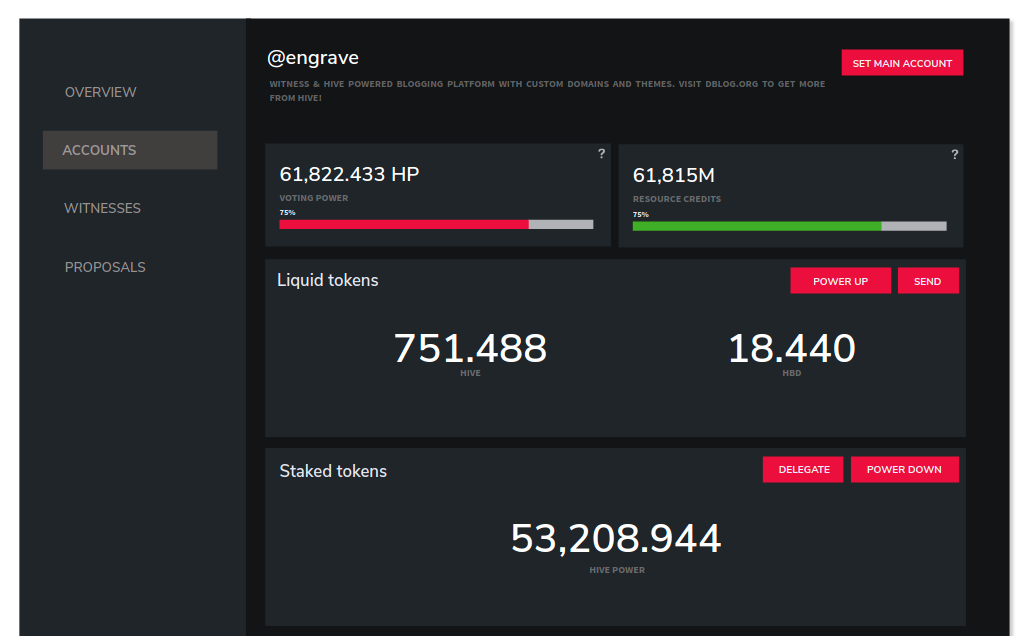
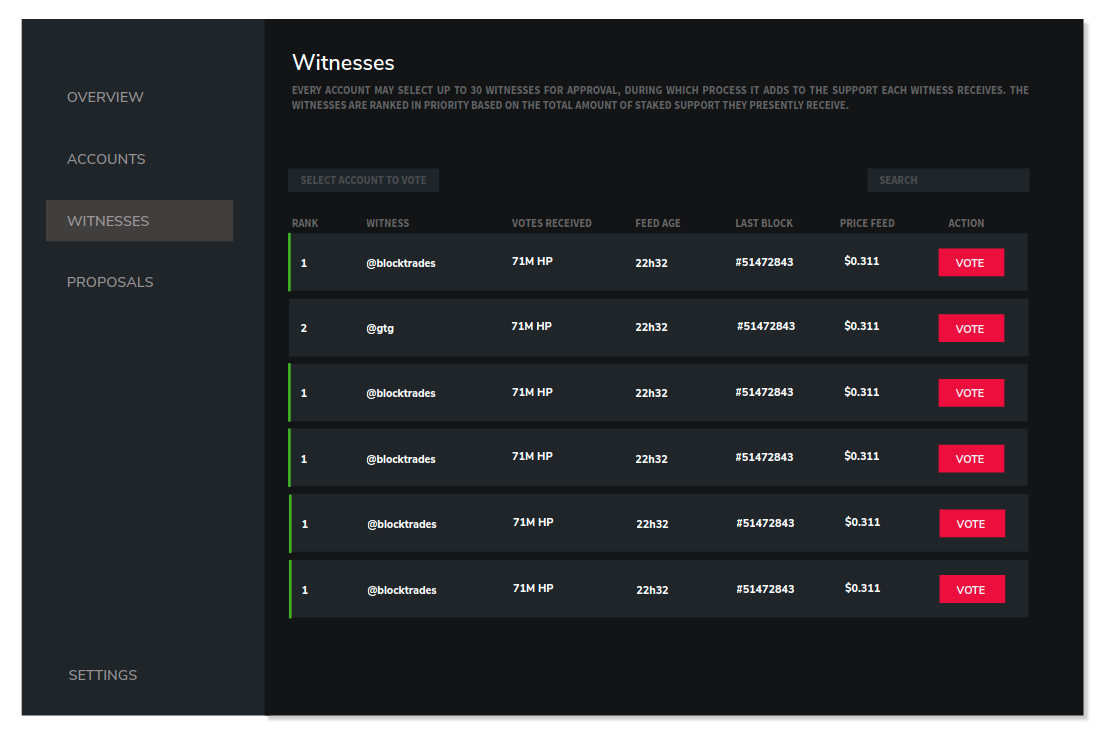
Follow me to be up to date with incoming development updates!
***
**Click on the image to vote for @engrave witness:**
[](https://hivesigner.com/sign/account-witness-vote?witness=engrave&approve=1)
</div>
See: JavaScript library for Ledger Nano S HIVE application by @engrave